How to Remove Special Characters and Dashes from Phone Number Input Fields in BigCommerce
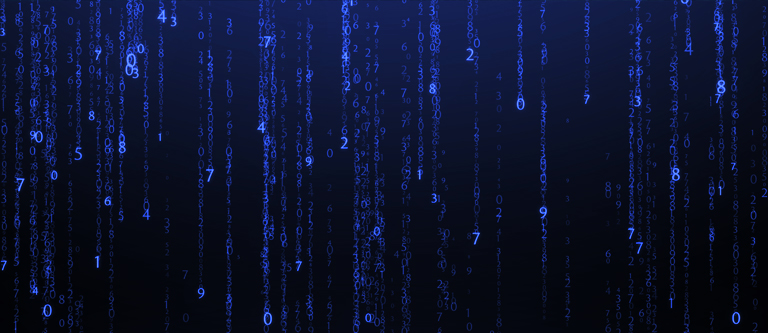
If you’re running an online store on BigCommerce, ensuring that your customer data is clean and consistent is crucial for smooth operations, especially when it comes to phone number fields. If you’re tired of seeing special characters, dashes, or spaces in your customers’ phone number inputs, you’re in the right place!
In this post, I’ll show you how to easily strip out any unwanted characters and make sure that only numbers are inputted into phone number fields in your BigCommerce store. The best part? We’ll use a simple script in BigCommerce’s Script Manager to handle it for you.
Step 1: What’s the Problem?
When customers enter their phone numbers in your store’s forms, they often include dashes, parentheses, or spaces (e.g., (123) 456-7890
). While this may look cleaner on the front end, it can cause issues with data formatting or integrations with third-party systems that expect the phone number to be in a standard format, like 1234567890
.
To solve this, we can strip out any special characters or dashes and ensure that only numbers are stored in the backend.
Step 2: The Script to Remove Special Characters
Here’s a simple jQuery script that will remove any non-numeric characters from phone number fields on your BigCommerce store’s account sign up and contact forms, and address fields during checkout. You can add this script to your store through Script Manager in BigCommerce.
The Code
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
$(document).on("keyup", "#phoneInput, #FormField_7_input, #account_phone, #contact_phone", function() {
var val_old = $(this).val();
var val = val_old.replace(/\D/g, ''); // Remove all non-digit characters
var len = val.length;
// Adjust the phone number format based on its length
if (len >= 1) val = val.substring(0); // Keep first number
if (len >= 3) val = val.substring(0, 4) + val.substring(4); // Add space after 3 digits
if (len >= 6) val = val.substring(0, 9) + val.substring(9); // Add space after 6 digits
if (len >= 10) val = val.substring(0, 15); // Limit to 10 digits
// Only update the input field if there was a change
if (val != val_old) $(this).focus().val('').val(val);
});
</script>
Step 3: How to Add the Script to BigCommerce
- Log in to your BigCommerce Admin Panel.
- Go to Script Manager: Navigate to Storefront > Script Manager.
- Add a New Script: Click on Create a Script.
- Choose the Placement: Select Footer as the placement for the script to run.
- Choose the Location: Select All pages as the location for the script to run on. This will ensure it runs on the checkout page as well.
- Choose the Script Category: Select Essential as the category for the script to run on.
- Enter the Script: Paste the script provided above into the Script section.
- Save the Script: After adding the script, hit Save.
This script will now run on any page that includes the phone number input fields defined by the IDs #phoneInput
, #FormField_7_input
, #account_phone
, or #contact_phone
. Feel free to change these IDs if your form uses different ones!
Step 4: Testing the Script
Once you’ve added the script, it’s important to test it:
- Go to the page with the phone number field: This might be your checkout page or account creation form.
- Try entering a phone number: Type a number with dashes, spaces, or parentheses (e.g.,
(555) 123-4567
). - Check the result: The phone number field should automatically strip out any non-numeric characters, leaving only the numbers.
Step 5: Customize for Your Store (Optional)
If your phone number fields use different IDs than the ones in the script, you can easily change them to match your own form’s field IDs. Just make sure the script listens for the correct input field by updating these selectors:
$(document).on("keyup", "#phoneInput, #FormField_7_input, #account_phone, #contact_phone", function() { ... });
For example, if your phone number field ID is #userPhone
, you would change the script to:
$(document).on("keyup", "#userPhone", function() { ... });
Final Thoughts
By adding this simple script to your BigCommerce store, you can ensure that phone numbers entered by your customers are formatted cleanly and consistently, without any special characters or spaces. This improves data accuracy and ensures a smoother experience for your backend systems.
Give it a try, and let me know if you run into any issues!